π Are you looking for ways to optimize Python code and boost performance? Python is widely used for web development, data science, and automation, but inefficient code can slow down execution. In this guide, we’ll explore five proven techniques to speed up Python and make your programs run faster.
π Table of Contents
1οΈβ£ Use Built-in Functions and Libraries
2οΈβ£ Use List Comprehensions Instead of Loops
3οΈβ£ Use Generators for Large Data Processing
4οΈβ£ Optimize Data Structures
5οΈβ£ Leverage Multi-threading and Multi-processing
π Conclusion
1οΈβ£ Use Built-in Functions and Libraries
One of the easiest ways to optimize Python code is to use built-in functions instead of writing custom implementations. These functions are written in C, making them significantly faster.
β
Example: Using sum()
Instead of a Loop
β Inefficient Code:
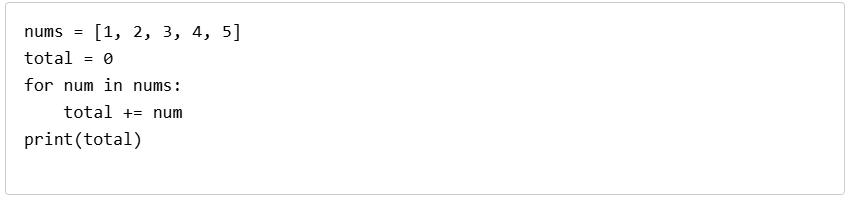
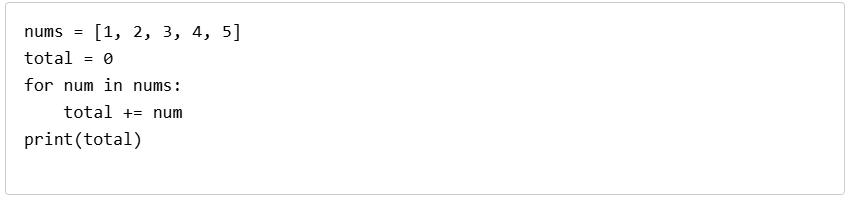
β Optimized Code:


β
sum()
is optimized in C and executes faster than a loop.
πΉ Reference: Learn more about Pythonβs built-in functions from the official documentation:
Python Built-in Functions
2οΈβ£ Use List Comprehensions Instead of Loops
List comprehensions are faster and more memory-efficient than traditional loops. They help speed up Python performance in operations involving lists.
β Example: Replace a Loop with List Comprehension
β Slow Loop Implementation:


β Optimized Code:


β This approach minimizes overhead and boosts execution speed.
πΉ Reference: Read more about list comprehensions on Real Python:
List Comprehensions in Python
3οΈβ£ Use Generators for Large Data Processing
Generators are memory-efficient because they yield values one at a time instead of storing them in memory. This is crucial when handling large datasets.
β Example: Using a Generator Instead of a List
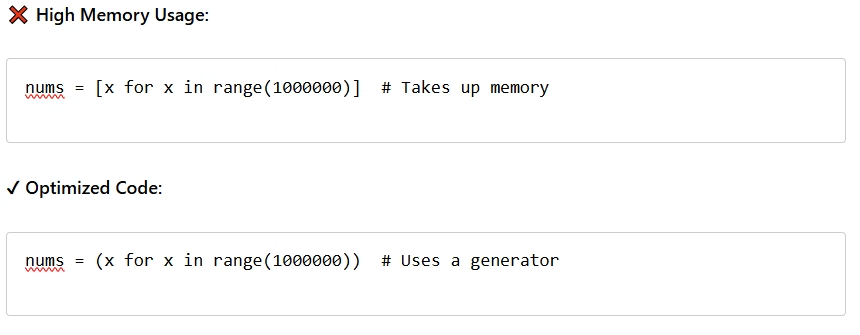
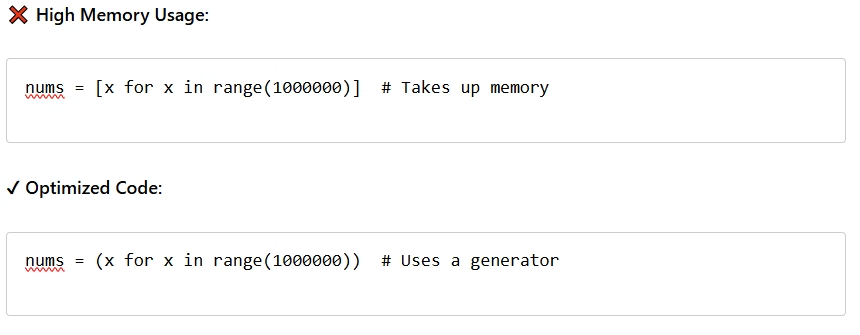
β The generator approach reduces RAM consumption.
πΉ Reference: Learn how Python generators work in detail from GeeksforGeeks:
Python Generators
4οΈβ£ Optimize Data Structures
Choosing the right data structure is key to improving Python performance.
β
Example: Using set()
for Fast Lookups
β Slow List Lookup (O(n)
)
names = ["Alice", "Bob", "Charlie"]
if "Bob" in names:
print("Found")
β Optimized Set Lookup (O(1)
)
names = {"Alice", "Bob", "Charlie"}
if "Bob" in names:
print("Found")
β Sets provide constant-time lookups, unlike lists that take linear time.
πΉ Reference: Read about Python data structures on W3Schools:
Python Data Structures
5οΈβ£ Leverage Multi-threading and Multi-processing
For CPU-bound tasks, use multiprocessing to take advantage of multiple cores. For I/O-bound tasks, use multithreading to improve efficiency.
β
Example: Using multiprocessing
for Parallel Execution
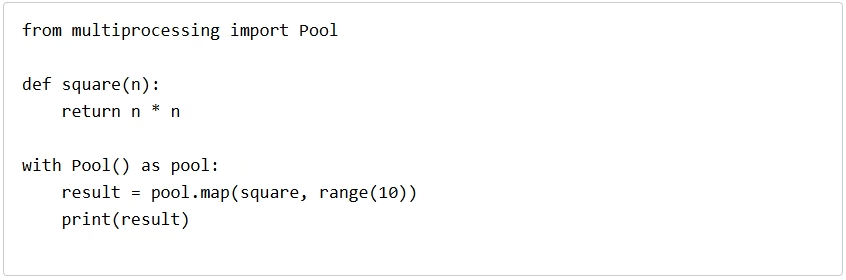
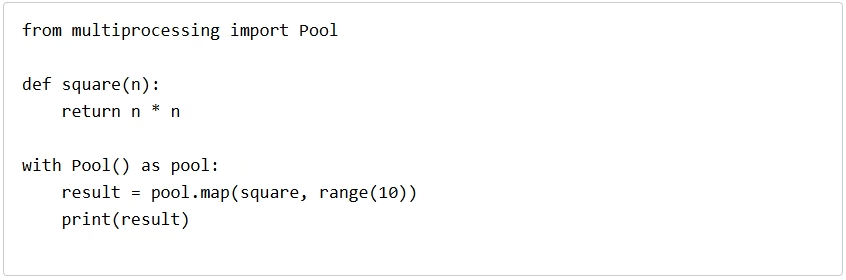
β
Pool.map()
parallelizes execution, significantly improving speed.
πΉ Reference: Learn about Python multiprocessing from Python Official Docs:
Python multiprocessing
π Conclusion
By following these five proven techniques, you can optimize Python code and achieve maximum performance:
β
Use built-in functions like sum()
, sorted()
, and map()
β
Replace loops with list comprehensions for efficiency
β
Use generators to handle large datasets without memory overload
β
Choose the right data structures (set
, deque
, defaultdict
)
β
Implement multi-threading/multiprocessing for faster execution
By implementing these strategies, you can speed up Python code, making your programs more efficient and scalable. π
π¬ Whatβs your favorite Python optimization technique? Share your thoughts in the comments!
FAQs for Optimize Python Code: 5 Proven Ways to Boost Performance
1. What does it mean to optimize Python code ?
2. Why is Python performance important for my project?
3. How can I optimize Python code using built-in functions?
sum()
, map()
, or sorted()
are implemented in C and are much quicker than looping through data manually.4. What are list comprehensions, and how do they improve Python performance?
5. How do generators help in optimize Python code?
6. What are some examples of optimize Python code using generators?
nums = (x for x in range(1000000)) # generator expression
This ensures lower memory usage as only one value is generated at a time.
7. How do I choose the right data structures to optimize Python code ?
O(1)
) compared to lists (O(n)
), and deque is optimized for faster append and pop operations.